Originally published at: Breakout Ball Game – BrainPad
Project Overview
Breakout Ball Game by DUE
Experience the exciting journey into the game domain with our DUE Breakout Ball Game. This project beautifully encapsulates the timeless excitement of the game development field, seamlessly integrating electronic circuits and microcomputers.
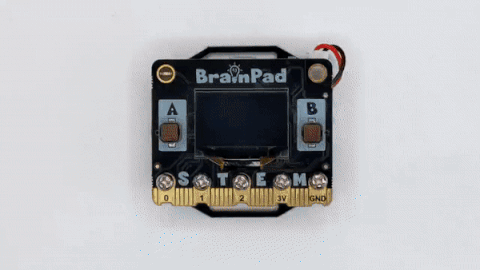
How It Works:
The Breakout game, a classic arcade favorite, puts players in control of a paddle, bouncing a ball against a wall of bricks. The goal? Break all the bricks while skillfully preventing the ball from escaping the screen.
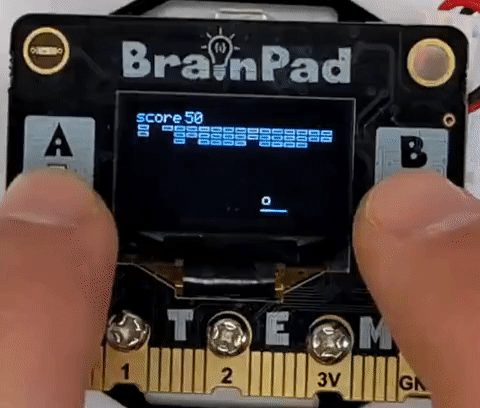
Hardware Requirements:
All you need is a BrainPad Pulse and a standard USB cable for a quick, hassle-free connection. Simply upload the provided code to the Pulse and you’re ready to go.
Software Requirements:
Executing this project requires zero installation. Just head to our user-friendly DUE console and let the magic unfold.
Code Overview:
Let’s break down the code into smaller steps and provide a comprehensive explanation for each part:
- Initialization (@init):
This section initializes various variables and arrays:
- c: Number of bricks.
- z: An array representing the existence of each brick (1 if present, 0 if destroyed).
- b: Array to store ball information (x, y, dx, dy).
- r: Radius of the ball.
- d: Diameter of the ball.
- x: Initial x-coordinate of the paddle.
- w: Width of the paddle.
- m: Paddle movement.
- A loop sets all elements of the z array to 1, indicating all bricks are initially present.
- Initializes the ball’s position (b[0] and b[1]) and velocity (b[2] and b[3]).
- Enables buttons ‘a’ and ‘b’ for input.
@init
c=48 # Brick count
dim z[c] # Bricks
dim b[4] # Ball x,y,dx,dy
r=2 # ball radius
d=r*2 # ball diameter
x=64-8 # Paddle X
w=16 # Paddle width
m=0 # Paddle movement
# Setup the bricks
for i in range(0,48):z[i]=1:next
# Setup ball
b[0]=64-r
b[1]=20
b[2]=1
b[3]=2
btnenable('a',1)
btnenable('b',1)
return
2. Start Game (@start):
This section resets the ball’s position and velocity:
- Sets the ball’s position above the paddle.
- Sets the ball’s direction based on its initial x-coordinate relative to the paddle.
- Displays “Ready” on the LCD and waits for one second before returning.
@start
# Reset ball
b[0]=64-r
b[1]=28
if b[0]>x
b[2]=-1
else
b[2]=1
end
lcdtext("Ready",1,52,30)
lcdshow();
wait(1000)
return
3. Main Game Loop (@loop):
This is the main game loop that handles the movement and collisions:
- Updates the ball’s position based on its velocity.
- Moves the paddle based on button inputs.
- Checks for collisions with the paddle, boundaries, and bricks.
- Updates the LCD display with the current state of the game.
- If all bricks are destroyed, it restarts the game.
s=0 # Score
init()
start()
@loop
# Move ball
b[0]=b[0]+b[2]
b[1]=b[1]+b[3]
# Move paddle
if btndown('a'):m=-2.5:end
if btndown('b'):m=2.5:end
if btnup('a') || btnup('b'):m=0:end
x=x+m
if x<0:x=0:end
if x>111:x=111:end
# Check missed paddle
if b[1]>60:start():end
# Check paddle collision
if b[1]>=60-r
if b[0]+r>=x && (b[0]-r)<=x+16
b[1]=60-r
b[2]=((b[0]-(x+8))/8)*2
b[3]=-b[3]
beep('p',100,20)
end
end
# Check boundary collision
if b[0]<=r || b[0]>=127-r:b[2]=-b[2]:end
if b[1]<=0:b[3]=-b[3]:end
# Check brick collision
if b[1]<=16 && b[1]>=10
u = b[0]
v = b[1]-10
i = trunc(u/8) + trunc(v/3)*16
if i>=0 && i<48
if z[i]
b[3]=-b[3]
z[i]=0
beep('p',100,20)
c=c-1
s=s+5
end
end
end
# Update screen
lcdclear(0)
u=0:v=0
for i in range(0,48)
u=(i%16)*8
v=10+trunc(i/16)*4
if z[i]
#lcdline(1, u+1, v, u+7, v)
lcdrect(1,u+1,v-1,7,3)
end
next
lcdline(1,x,60,x+16,60)
lcdcircle(1, b[0], b[1], r)
lcdtext("score",1,0,0)
lcdtext(str(s),1,32,0)
lcdshow()
if c=0
# Restart if we got all the bricks
init()
start()
end
goto loop
@start
# Reset ball
b[0]=64-r
b[1]=28
if b[0]>x
b[2]=-1
else
b[2]=1
end
lcdtext("Ready",1,52,30)
lcdshow();
wait(1000)
return
@init
c=48 # Brick count
dim z[c] # Bricks
dim b[4] # Ball x,y,dx,dy
r=2 # ball radius
d=r*2 # ball diameter
x=64-8 # Paddle X
w=16 # Paddle width
m=0 # Paddle movement
# Setup the bricks
for i in range(0,48):z[i]=1:next
# Setup ball
b[0]=64-r
b[1]=20
b[2]=1
b[3]=2
btnenable('a',1)
btnenable('b',1)
return
Customization:
- Enhance Game Ending:
We’ve left the game without a fixed ending on purpose. Why? Because we’re handing the reins to you! So, we are looking forward to seeing your own way endgame that suits your style like losing lives or reaching to specific score.
- Explore Different Programming Languages with BrainPad Pulse:
You can create the same game using alternative languages such as Python, C#, JavaScript, and more. Discover the various coding options available here.